Club.noww.in
Python's Boolean operators are used for combining Boolean expressions and negating Boolean expressions.
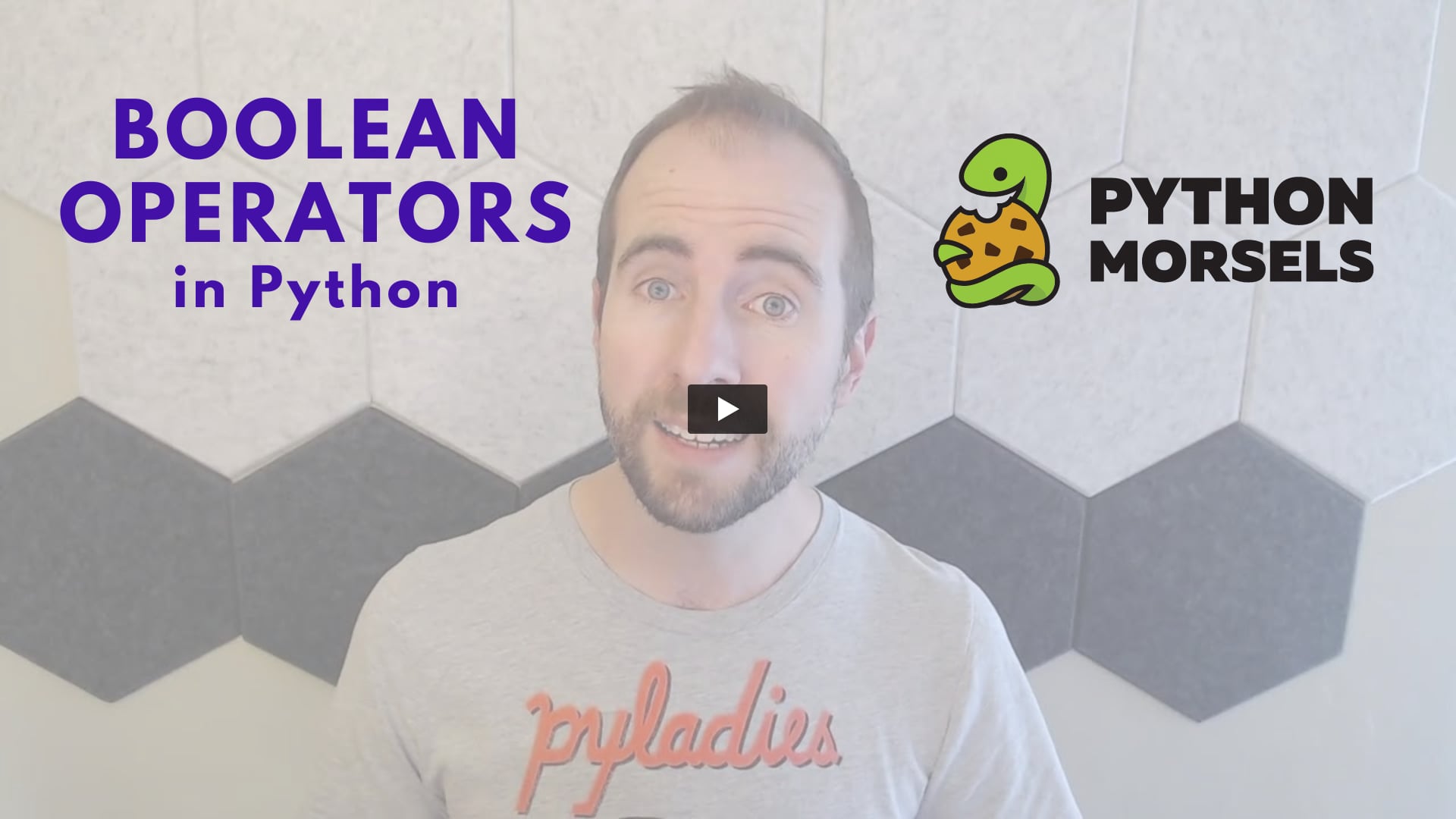
Table of contents
Combining two if statements using and
Here we have a program called word_count.py:
This program has an if statement that checks whether we've written enough words each day, with the assumption that we need to write 50,000 words every 30 days.
If our word count is under 1,666 words (50,000 / 30) it will say we need to write more:
We'd like to modify our if condition to also make sure that we only require this if today's date is in the month of November.
We could do that using Python's datetime module:
That is_november variable will be True if it's November and False otherwise:
If we combine this with the code we had before, we could use two if statements:
One of our if statements checks whether we're under our word limit. The other if statement checks whether it's the month of November. If both are true then we end up printing out that we still need to write more words. Otherwise we print a success message:
This works, but there is a better way to write this code.
We could instead use Python's and operator to combine these two conditions into one:
We're using a single if statement to asking whether it's November and whether our word count is less than we expect.
Combining expressions with Boolean operators
Python's and operator is a …